typedef struct tagACI_ProgOption_Params
{
UINT Size; // (in) Size of structure, in bytes
LPCSTR OptionName; // (in) Name of the option. For lists, it should be in the form "List array name^List Name", e.g. "Configuration Bits^Oscillator"
CHAR Units[32]; // (out) Option measurement units ("kHz", "V", etc.)
CHAR OptionDescription[64]; // (out) Description of the option
CHAR ListString[64]; // (out) For ACI_PO_LIST option: Option string for Value.ListIndex
UINT OptionType; // (out) Option type: see ACI_PO_xxx constants
BOOL ReadOnly; // (out) Option is read-only
union // (in || out) Option value
{
LONG LongValue; // (in || out) Value for ACI_PO_LONG option
FLOAT FloatValue; // (in || out) Value for ACI_PO_FLOAT option
LPSTR String; // (in || out) Pointer to string for ACI_PO_STRING option
ULONG CheckBoxesValue; // (in || out) Value for ACI_PO_CHECKBOXES option
UINT StateIndex; // (in || out) State index for ACI_PO_LIST option
LPBYTE Bitstream; // (in || out) Pointer to bitstream data for ACI_PO_BITSTREAM option
} Value;
UINT VSize; // For ACI_SetProgOption():
// in: Size of Bitstream if OptionType == ACI_PO_BITSTREAM
// For ACI_GetProgOption():
// in: Size of buffer pointed by Bitstream if OptionType == ACI_PO_BITSTREAM
// in: Size of buffer pointed by String if OptionType == ACI_PO_STRING
// out: Size of buffer needed for storing Bitstream data if OptionType == ACI_PO_BITSTREAM.
// Set Value.Bitstream to NULL to get buffer size without setting the bitstream data
// out: Size of buffer needed for storing String if OptionType == ACI_PO_STRING, including the terminating NULL character.
// Set Value.String to NULL to get buffer size without setting the string
UINT Mode; // (in) For ACI_SetProgOption(): SEE ACI_PP_MODE_... constants
} ACI_ProgOption_Params;
OptionName
|
The name of the programming option - for example "Vcc". For the ACI_PO_LIST - type options, where the options are grouped into a list, you should specify both the list name and the option name in the following way: <List name>^<Option name> (For example, Configuration_bits^Generator. There are no restrictions on use of uppercase and lowercase characters in the option names.
|
Units
|
After executing the ACI_GetProgOption function this structure member returns an abbreviation of the units, in which the programmer represents or measures the OptionName. For example, for the Vcc structure member, Units = "V".
|
OptionDescription
|
After executing the ACI_GetProgOption function this structure member returns the option description.
|
ListString
|
After executing the ACI_GetProgOption function for the ACI_PO_LIST - type options this structure member returns a string that describes the current option's value or status. For example, XT - Standard Crystal for the option Configuration bits^Generator.
|
OptionType
|
After executing the ACI_GetProgOption function this structure member returns the option's presentation format - for example: integer, floating point, list, bitstream, etc.. See the ACI_PO_xxx* constant description in the aciprog.h header file below.
|
ReadOnly
|
Setting ReadOnly=TRUE disables modification of the option specified by the ACI_GetProgOption function.
|
Value
|
Use of this union depends on the ACI_PO_LONG* option type as it is shown in the matrix below:
Option type
|
Use of the Value union
|
ACI_PO_LONG
|
The option is in the Value.LongValue
|
ACI_PO_FLOAT
|
The option is in the Value.FloatValue
|
ACI_PO_STRING
|
The option is represented as a string, the pointer on which is in the Value.String. See the note below.
|
ACI_PO_CHECKBOXES
|
The option represents a 32-bit integer word, in which you can individually toggle each bit that represents a particular flag in the option setting dialog. The option is in the Value.CheckBoxesValue. See, for example, the Fuse setting dialog for the ATtiny45 MCU implemented as an array of check boxes.
|
ACI_PO_LIST
|
It represents a list of alternative choices. Only one of them can be selected at a time, so the parameter changes its value in a range 0, 1, 2 to N. The option is in the Value.CheckStateIndex. See, for example, the Oscillators setting dialog for the PIC12F509 MCU implemented as an alternatively chosen radio buttons
|
ACI_PO_BITSTREAM
|
Stream of bits. This option type is not in use yet but can be used for future applications.
|
|
VSize
|
Size of the buffer assigned for storing the string if the option type is the ACI_PO_STRING. See the note below.
|
Mode
|
Mode of using of the structure member Value (See the description of the ACI_PP_xxx** constants in the aciprog.h<) header file:
The Mode setting (value)
|
Use of the parameter Value
|
ACI_PP_MODE_VALUE
|
1) For measuring (getting): use the Value in order to get an actual Option value;
2) For setting: use the Value to set a particular Option value.
|
ACI_PP_MODE_DEFAULT_VALUE
|
1) If used with the ACI_GetProgOption function it issues a command to put the default Option value into the Value.
2) If used with the ACI_SetProgOption function, the Value will be ignored; the Option will be set to the default level defined in the ChipProg hardware.
|
ACI_PP_MODE_MIN_VALUE
|
1)If used with the ACI_GetProgOption function it commands to put the minimal Option value into the Value.
2) If used with the ACI_SetProgOption function the Value will be ignored; the Option will be set to the minimal level defined in the ChipProg hardware, if it is possible for the Option of this type.
|
ACI_PP_MODE_MAX_VALUE
|
1) If used with the ACI_GetProgOption function it commands to put the maximal Option value into the Value.
2) If it is used with the ACI_SetProgOption function the Value will be ignored; the Option will be set to the maximal level defined in the ChipProg hardware, if it is possible for the Option of this type.
|
|
This is the bit definition from the aciprog.h header file:
*// ACI Programming Options defines
#define ACI_PO_LONG 0 // Signed integer option
#define ACI_PO_FLOAT 1 // Floating point option
#define ACI_PO_STRING 2 // String option
#define ACI_PO_CHECKBOXES 3 // 32-bit array of bits
#define ACI_PO_LIST 4 // List (radiobuttons)
#define ACI_PO_BITSTREAM 5 // Bit stream - variable size bit array
**// ACI Programming Option Mode constants for ACI_GetProgOption()/ACI_SetProgOption()
#define ACI_PP_MODE_VALUE 0 // Get/set value specified in Value member of the ACI_ProgOption_Params structure
#define ACI_PP_MODE_DEFAULT_VALUE 1 // Get/set default option value, ignore Value member
#define ACI_PP_MODE_MIN_VALUE 2 // Get/set minimal option value, ignore Value member
#define ACI_PP_MODE_MAX_VALUE 3 // Get/set maximal option value, ignore Value member
Note for use of the ACI_GetProgOption:
In order to get the buffer size necessary for storing the Option ACI_PO_STRING, you should make the first call of the ACI_GetProgOption function with the Value.String= NULL. Then the function will return the VSize equal to the buffer size, including zero at the string's end. In your program, assign the buffer of this size, put the Value.String into the buffer pointer and call the ACI_GetProgOption again.
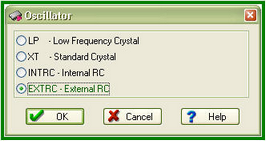
See also: ACI_GetProgOption, ACI_SetProgOption
|